Hosting External JS files locally can help speed up your site in a lot of ways. It will help reducing extra DNS lookups &load them faster using a single HTTP/2 connection. Hosting external files locally in WordPress will also resolve the “leverage browser caching” issue in google page speed insight as well.
Serving these files from your own server gives you complete control over the caching and compression settings of those files, by which you can control how long those files stay on visitors’ browser cache & compress them using Gzip or Brotli.
In this guide, I am going to show how to store facebook pixel JS locally. But the steps will be the same for any kind of file.
Downloading the file locally
First things first we need to figure out which file we need to download ($remoteFile
). Where you are going to save the file ($uploadDir
) and the name of the file with the extension($localFile
)
Let’s analyze the code first and Identify what we need to download, Here is sample Facebook Pixel code-
<!-- Facebook Pixel Code -->
<script>
!function(f,b,e,v,n,t,s)
{if(f.fbq)return;n=f.fbq=function(){n.callMethod?
n.callMethod.apply(n,arguments):n.queue.push(arguments)};
if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0';
n.queue=[];t=b.createElement(e);t.async=!0;
t.src=v;s=b.getElementsByTagName(e)[0];
s.parentNode.insertBefore(t,s)}(window, document,'script',
'https://connect.facebook.net/en_US/fbevents.js');
fbq('init', '50852268307XXXX');
fbq('track', 'PageView');
</script>
<!-- End Facebook Pixel Code -->
In this file the external file is http://connect.facebook.net/en_US/fbevents.js
Now we need to edit and create a PHP file in your server. Replace the $remoteFile with the file, you want to download, $localFile the path where to save the download file & $uploadDir
with the path of the folder where it will be saved.
<?php
// Script to download and update any js-file, Credits go to: Matthew Horne
$remoteFile = 'http://connect.facebook.net/en_US/fbevents.js';
$localFile = 'local/file/will/be/saved/here/local_fb.js';
// Check if directory exists, otherwise create it.
$uploadDir = 'local/file/will/be/saved/here/';
if (!file_exists($uploadDir)) {
mkdir($uploadDir);
}
// Connection time out
$connTimeout = 10;
$url = parse_url($remoteFile);
$host = $url['host'];
$path = isset($url['path']) ? $url['path'] : '/';
if (isset($url['query'])) {
$path .= '?' . $url['query'];
}
$port = isset($url['port']) ? $url['port'] : '80';
$fp = @fsockopen($host, $port, $errno, $errstr, $connTimeout );
if(!$fp){
// On connection failure return the cached file (if it exist)
if(file_exists($localFile)){
readfile($localFile);
}
} else {
// Send the header information
$header = "GET $path HTTP/1.0\r\n";
$header .= "Host: $host\r\n";
$header .= "User-Agent: Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.6) Gecko/20070725 Firefox/2.0.0.6\r\n";
$header .= "Accept: */*\r\n";
$header .= "Accept-Language: en-us,en;q=0.5\r\n";
$header .= "Accept-Charset: ISO-8859-1,utf-8;q=0.7,*;q=0.7\r\n";
$header .= "Keep-Alive: 300\r\n";
$header .= "Connection: keep-alive\r\n";
$header .= "Referer: http://$host\r\n\r\n";
fputs($fp, $header);
$response = '';
// Get the response from the remote server
while($line = fread($fp, 4096)){
$response .= $line;
}
// Close the connection
fclose( $fp );
// Remove the headers
$pos = strpos($response, "\r\n\r\n");
$response = substr($response, $pos + 4);
// Return the processed response
echo $response;
// Save the response to the local file
if(!file_exists($localFile)){
// Try to create the file, if doesn't exist
fopen($localFile, 'w');
}
if(is_writable($localFile)) {
if($fp = fopen($localFile, 'w')){
fwrite($fp, $response);
fclose($fp);
}
}
}
?>
Now upload the PHP file somewhere in the server via FTP.
Now SSH to it by using the command-
php -f /path//where/you/uploaded/your/fb_pixel_download.php
If everything is perfect you will see a new file named local_fb.js created in the upload directory.
Setting up a cronjob to update the file periodically.
To setup cronjob, at first go to Crontab Generator and select the following options, This will run the script every 6 hours. you may change according to your needs.
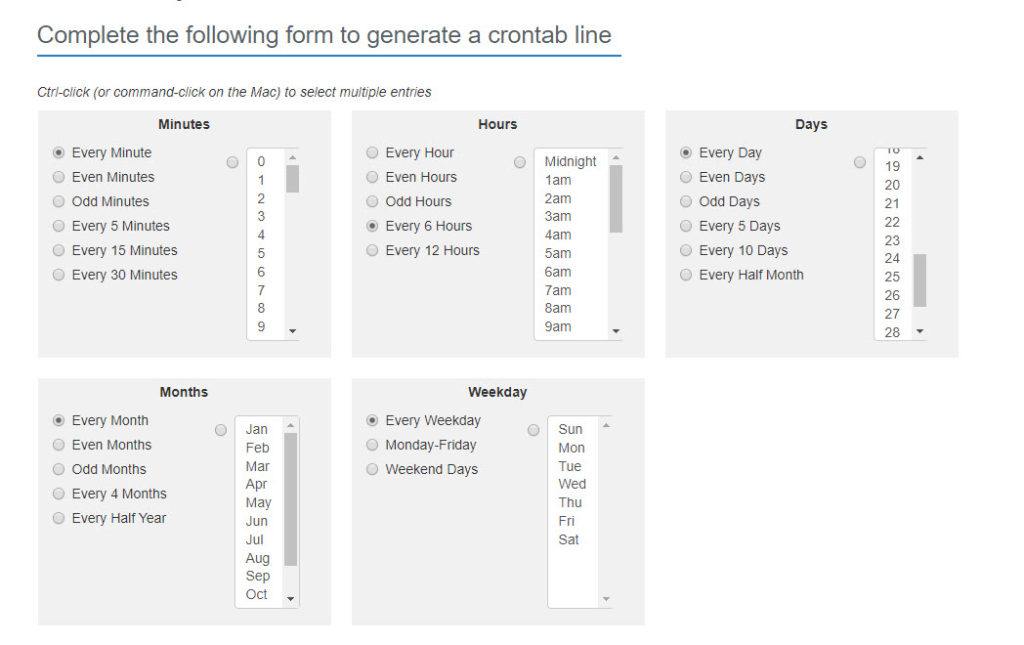
Now in command to execute option put the command below & hit generate.
/usr/bin/php -f /path/to/your/script.php
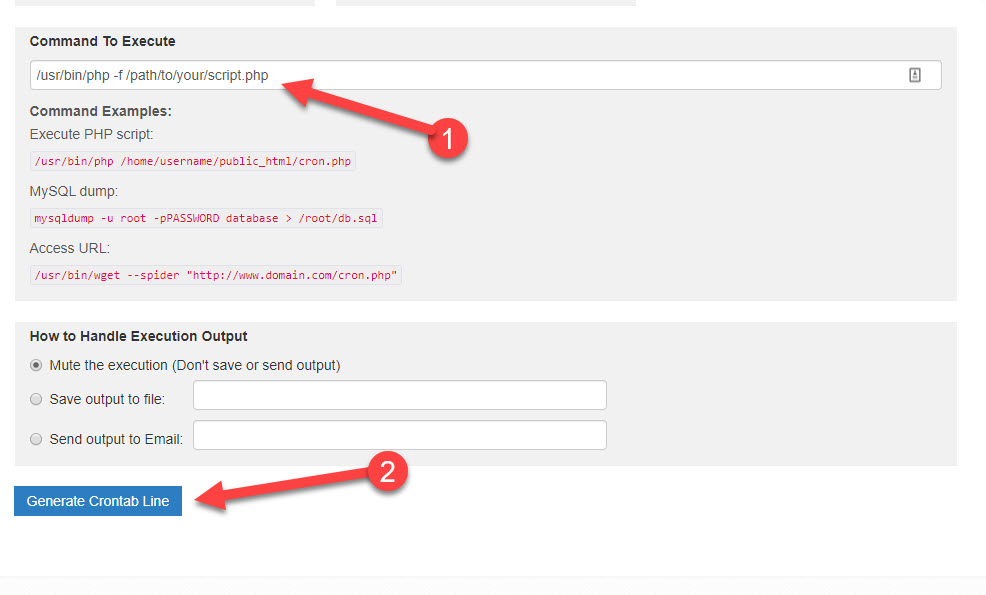
Now it will generate a cron command like this copy it to clipboard.
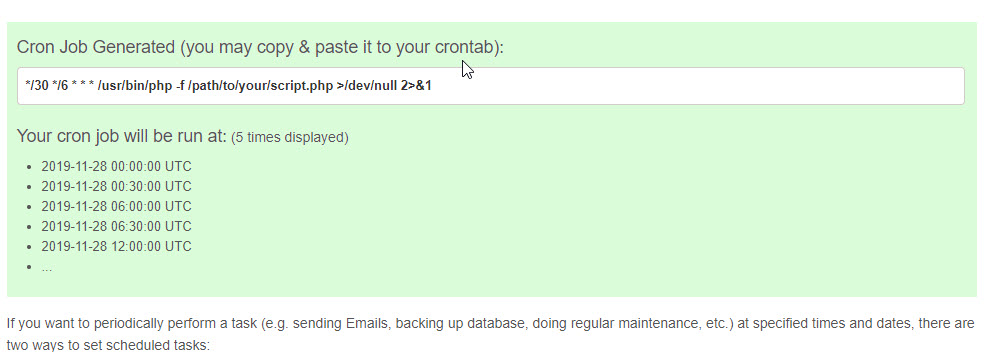
Now SSH into your server and type the command below-
crontab -e
Then it will ask you to choose the editor type. Choose 1. The output should like this.
[simterm]# crontab -e
no crontab for root – using an empty one
Select an editor. To change later, run ‘select-editor’.
1. /bin/nano <---- easiest
2. /usr/bin/vim.basic
3. /usr/bin/vim.tiny
4. /bin/ed>Choose 1-4 [1]: 1
[/simterm]
Now paste the cron command in the end. Then save it using CTRL+X, then type Y and then Enter. Now you are done!
Updating The final code
Now we need to change the URLs within the provided javascript snippets. For facebook pixel this how it should look this.
Before
<!-- Facebook Pixel Code -->
<script>
!function(f,b,e,v,n,t,s)
{if(f.fbq)return;n=f.fbq=function(){n.callMethod?
n.callMethod.apply(n,arguments):n.queue.push(arguments)};
if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0';
n.queue=[];t=b.createElement(e);t.async=!0;
t.src=v;s=b.getElementsByTagName(e)[0];
s.parentNode.insertBefore(t,s)}(window, document,'script',
'https://connect.facebook.net/en_US/fbevents.js');
fbq('init', '50852268307XXXX');
fbq('track', 'PageView');
</script>
<!-- End Facebook Pixel Code -->
After
<!-- Facebook Pixel Code -->
<script>
!function(f,b,e,v,n,t,s)
{if(f.fbq)return;n=f.fbq=function(){n.callMethod?
n.callMethod.apply(n,arguments):n.queue.push(arguments)};
if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0';
n.queue=[];t=b.createElement(e);t.async=!0;
t.src=v;s=b.getElementsByTagName(e)[0];
s.parentNode.insertBefore(t,s)}(window, document,'script',
'https://yoursite.com/local/file/will/be/saved/here/local_fb.js');
fbq('init', '50852268307XXXX');
fbq('track', 'PageView');
</script>
<!-- End Facebook Pixel Code -->
Conclusion
In theory, everything should work, but make sure you cross-check if it is working by using a different browser or incognito window. If everything works. Then cheers, You are hosting External File Locally In WordPress! You can repeat this step as much as you want for multiple files, because, by doing this you are minimizing DNS requests, which is better for your sites’ loading speed. If something doesn’t work, let me know via comments. I will try to help, If possible.
Thank you for the detailed article, managed to host 5 external files locally!
Thank You Clone!
This is the way.
BTW, 3 months pass,
Is it work fine? my bro.
Yes, Bone,
It works fine
Upload a .js file and test the file
Ex. http://Www.test.com/test/new.js
Now after some time update this file but browser load old file
Than delete
http://Www.test.com/test/new.js this file,clear cache, plugin cache
This file not show my cpnal but open on any browser
I don’t no what to do
Now after 2 days search Google but not find any solution please help me
Sorry couldn’t understand your query, can you email me or send me a message on Facebook?
I am facing an issue, I am using shared hosting and i don’t know where to upload that php file.
Can you help me out? How can i figure out the correct directory to upload this file…
Looking forward to your positive response.
Thanks
This is awesome! Is there an existing plugin for this?